GPS Location Tags
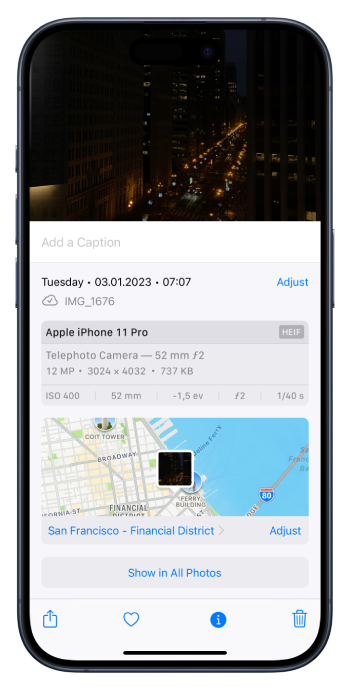
What are GPS Location Tags?​
GPS Location Tags are location properties stored in image files (via EXIF tags) or video files (via QuickTime/MP4 tags).
VisionCamera provides an API to easily add such location tags to captured photos or videos.
Configure Location Permissions​
First, you need to add the required permissions to access the user's location:
- React Native
- Expo
iOS​
Open your project's Info.plist
and add the following lines inside the outermost <dict>
tag:
<key>NSLocationWhenInUseUsageDescription</key>
<string>$(PRODUCT_NAME) needs access to your location.</string>
Android​
Open your project's AndroidManifest.xml
and add the following lines inside the <manifest>
tag:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
Managed Expo​
Enable the enableLocation
property inside your app's Expo config (app.json
, app.config.json
or app.config.js
):
{
"name": "my app",
"plugins": [
[
"react-native-vision-camera",
{
// ...
"enableLocation": true,
"locationPermissionText": "[my app] needs your location."
}
]
]
}
Finally, compile the mods:
npx expo prebuild
To apply the changes, build a new binary with EAS:
eas build
Request Location Permissions​
After adding the required permissions to your app's manifests, prompt the user to grant location permission at runtime:
- Hooks API
- Imperative API
Get or request permissions using the useLocationPermission
hook:
const { hasPermission, requestPermission } = useLocationPermission()
Get the current permission status:
const permissionStatus = Camera.getLocationPermissionStatus()
And if it is not granted
, request permission:
const newPermissionStatus = await Camera.requestLocationPermission()
Enable GPS Location Tags​
Use the enableLocation
property to start streaming location updates and automatically add GPS Location Tags to images (EXIF tags) and videos:
<Camera {...props} enableLocation={true} />
Once enabled, all captured photos (see "Taking Photos") and videos (see "Recording Videos") will contain location tags.
Enable or disable Location APIs​
Location APIs are considered privacy-sensitive APIs by Apple. When you are not using privacy-sensitive APIs (like location) but still include them in your app's code, the Apple review team might reject your app.
To avoid that, you can disable the location APIs and VisionCamera will not compile those privacy-sensitive APIs into your app. Simply set the required flag before building your app:
- React Native
- Expo
Inside your Podfile
, add the VCEnableLocation
flag and set it to false
:
$VCEnableLocation = false
Inside your Expo config (app.json
, app.config.json
or app.config.js
), add the enableLocation
flag to the react-native-vision-camera
plugin and set it to false
:
{
"name": "my app",
"plugins": [
[
"react-native-vision-camera",
{
// ...
"enableLocation": false
}
]
]
}
Then rebuild your app and any location-related APIs will be excluded from the build.